Spring Boot Authentication and Authorization
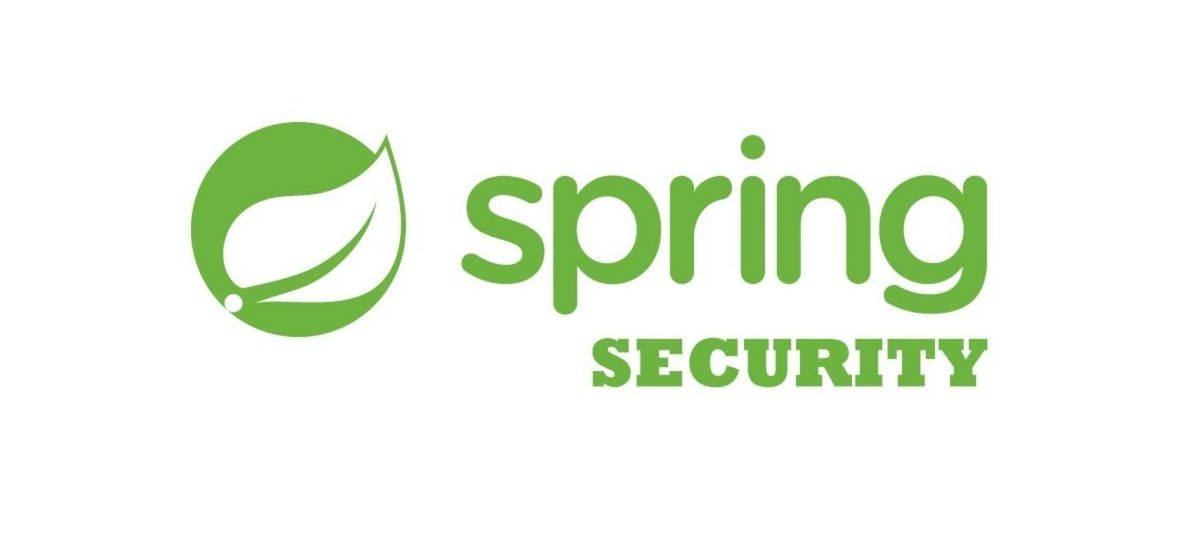
Spring Boot is an open source Java-based framework that is widely used to create enterprise level microservices. Implementing secure authentication and authorization in such microservices brings up a new set of challenges to developers. Spring Security comes in handy by providing a powerful, secure and customizable authentication and authorization framework.
Spring Security makes use of a Role Based Access Control (RBAC) model to help mitigate some of the inherent Authentication and Authorization security threats such as violation of least privilege principle, insecure direct object references and unauthorized privilege escalation.
Authentication and Authorization
Authentication is the process of ascertaining the identity of user that wants to access a certain resource. Spring Security supports a wide range of authentication methods including:-
- HTTP BASIC authentication through username and password
- LDAP (Lighweight Directory Access Protocol)
- Form-based authentication
- OpenID authentication
Authorization on the other hand refers to the process of verifying what specific applications, files, and data the authenticated user has access to. Spring Security supports authorization through JSON Web Token (JWT) and OAuth2.
Implementation
To illustrate implementation of Spring Security is a Spring Boot application, we are going to create a simple service that supports user registration, login, generation of access and refresh token and posting and viewing of articles based on granted privileges. This will be supported by a MySQL database.
Spring Security is added into Spring Boot by including the following maven configuration in the pom.xml file. The latest version can be found on Maven Central.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
The application flow is as illustrated below for authentication, authorization and generation of refresh token.
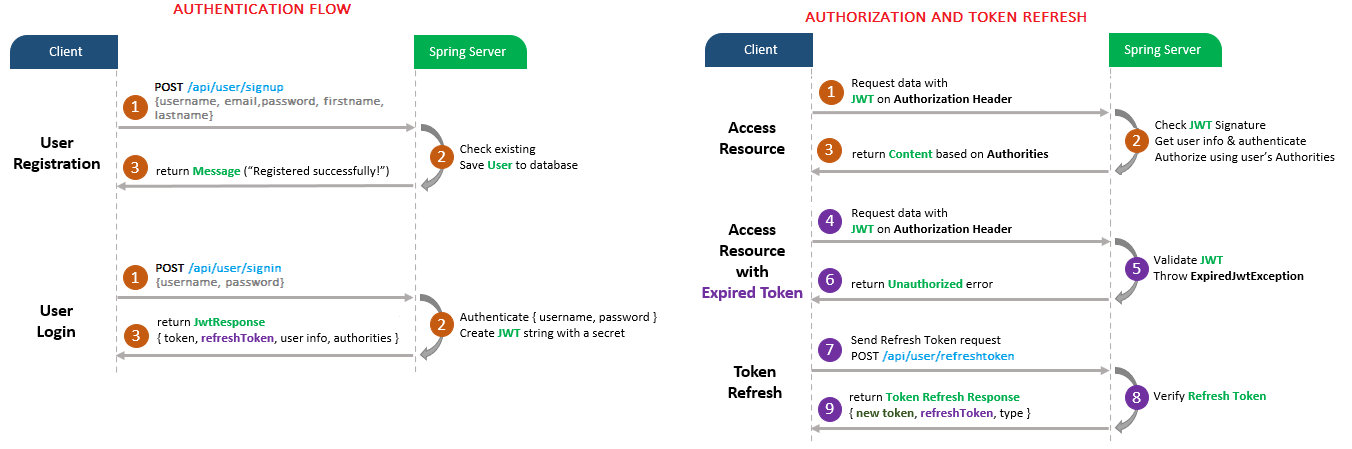
On signin, access and refresh tokens are generated. The access token is then used to access or manage resources e.g. add articles and manage users until its expiry where the refresh token can be used to generate a new access token.
The APIs provided are as below.
Method | URL | Role Required | Action |
---|---|---|---|
POST | /api/user/signup | NONE | User register |
POST | /api/user/signin | NONE | User login |
POST | /api/user/refreshtoken | ALL ROLES | User get refresh token |
POST | /api/user/signout | ALL ROLES | User sign out |
POST | /api/res/article/add | ROLE_USER ROLE_MODERATOR | Post article |
GET | /api/res/articles | NONE | View all articles |
GET | /api/res/article/{id} | NONE | View article by Id |
POST | /api/res/user/add | ROLE_ADMIN | Add user |
GET | /api/res/users | ROLE_ADMIN | View all users |
GET | /api/res/user/profile | ALL ROLES | Vie own profile |
Download the full source code and postman collection to test the API endpoints.