Securing Android Application User Sessions
Android application developers grapple with implementing user sessions that provide seamless user experience without compromising on application security. Android framework provides the option to use SharedPreferences, which is an easy and efficient way to store a small amount of key-value data especially for persisting user sessions. SharedPreferences however store data as plain text and therefore not ideal while storing sensitive data such as access keys and passwords as well as Personally Identifiable Information (PII).
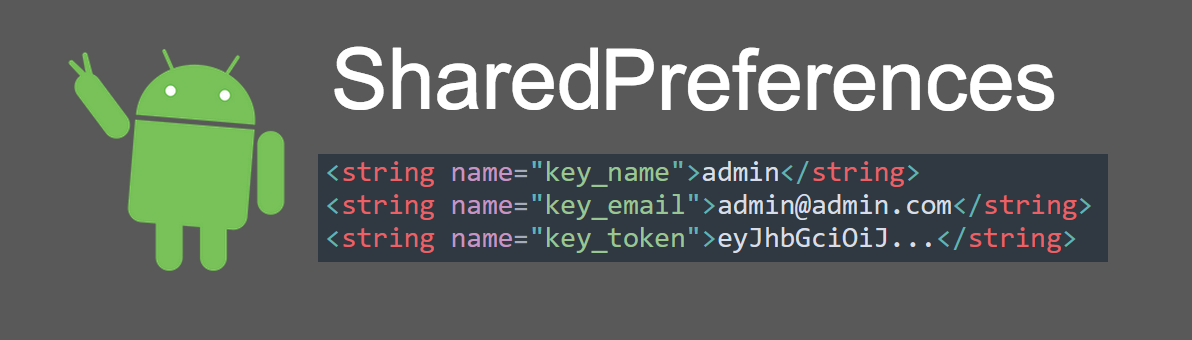
OWASP Mobile Top 10 highlights Insecure Data Storage as one of the major security issues affecting mobile applications. This is noted to be attributed to, but is not limited to, binary and XML data stores such as SharedPreferences. The AndroidX Security library was recently released to support encryption of SharedPreferences for applications with a minimum SDK version 23.
Encrypted SharedPreferences
Encrypted SharePreferences are bundled within the AndroidX security library which can be added into an application through gradle (app/build.gradle) as follows:-
dependencies {
...
implementation "androidx.security:security-crypto:1.1.0-alpha03"
...
}
Secure User Sessions
Encrypted SharedPreferences can be used in securing user sessions by storing the session data such as access tokens, keys, passwords and PII data in encrypted key-value pairs. This data is stored in the user device and can be retrieved any time when its required from the path below.
data/data/<YOUR_APP_ID>/shared_prefs/<SHARED_PREF_NAME>.xml
To illustrate the implementation of encrypted SharedPreferences in securing user session data, I have created a simple Android application that allows a user to register (1), login (2) and view their profile data as retrieved from the stored data in SharedPreferences (3).
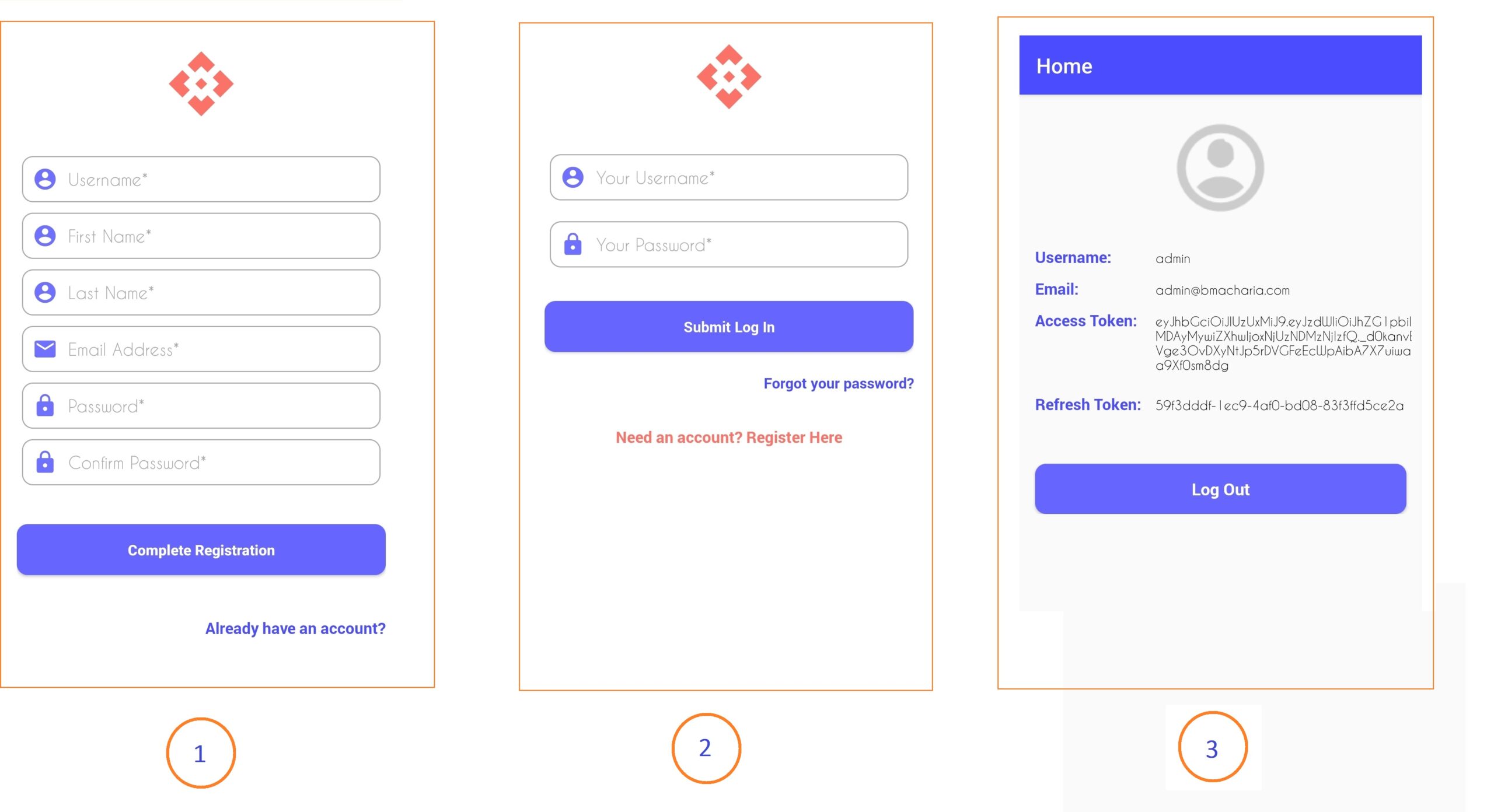
When the user successfully logs in, their username, email, role, access token and refresh token is stored in the SharedPreferences file as illustrated below.
public class SharedPrefManager {
// SharedPreferences Encryption method
public SharedPreferences getEncryptedSharedPreferences() {
MasterKey masterKey = null;
masterKey = new MasterKey.Builder(mCtx, MasterKey.DEFAULT_MASTER_KEY_ALIAS)
.setKeyScheme(MasterKey.KeyScheme.AES256_GCM)
.build();
SharedPreferences sharedPreferences = null;
sharedPreferences = EncryptedSharedPreferences.create(
mCtx,
USER_SHARED_PREF_NAME,
masterKey,
EncryptedSharedPreferences.PrefKeyEncryptionScheme.AES256_SIV,
EncryptedSharedPreferences.PrefValueEncryptionScheme.AES256_GCM);
return sharedPreferences;
}
//Method to store user data in shared preferences after login
public void userLogin(User user) {
SharedPreferences.Editor editor = getEncryptedSharedPreferences().edit();
editor.putString(KEY_ID, user.getUserId());
editor.putString(KEY_USERNAME, user.getUsername());
editor.putString(KEY_EMAIL, user.getEmail());
editor.putString(KEY_ROLE, user.getRole());
editor.putString(KEY_ATOKEN, user.getAccessToken());
editor.putString(KEY_RTOKEN, user.getRefreshToken());
editor.apply();
}
}
The Advanced Encryption Standard with 256 bits (AES-256) is used to encrypt the SharedPreferences key-value data as shown below.
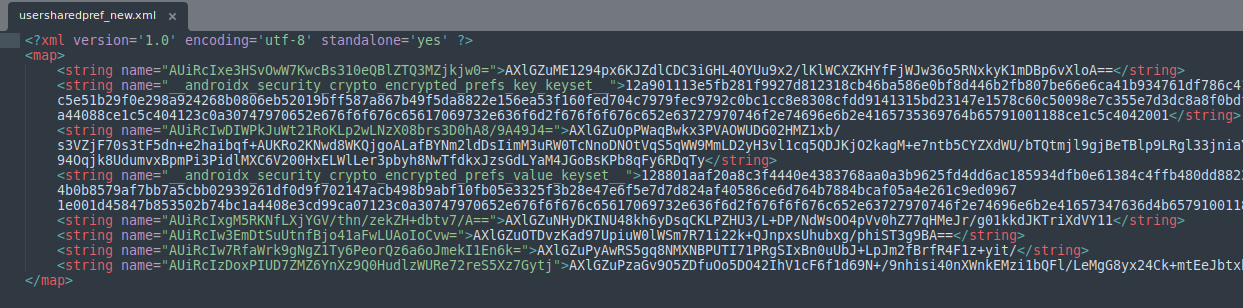
The application source code, APK file and sample encrypted SharedPreferences file can be downloaded here: – Download
Encrypted SharePreferences can be used a secondary layer of protection after code obfuscation with security tools such as Android ProGuard in protecting secrets within Android applications.